Javascript should be placed in footer of html document wherever possible. For libraries like jQuery we don’t often have a choice as there may be some other javascript code which depends on that. But we should design site in such a way that maximum javascript code is placed in the end.
Reason for placing Javascript in footer
Javascript execution blocks rendering of html page as there may be css style and html creation/edit code in javascript. So browser waits till the whole execution of that javascript is over before rendering subsequent html.
Experiment for Javascript in header vs footer
We’ll do a simple experiment where we’ll place the following javascript code in page header in one case and footer in another case.
/*jslint indent: 2 */ "use strict"; (function fake_sleep(millis) { var startTime = new Date(), currentTime = new Date(); while (currentTime - startTime < millis) { currentTime = new Date(); } }(500));
Case 1 – Javascript in header
Here is the sample code which puts javascript slow.js in html page header which causes a delay of 500 milliseconds. Then we print the time difference at the end of the html document using javascript.
<!DOCTYPE html> <html> <head> <title>Javascript in header example</title> <script type="text/javascript"> var startTime = new Date(); </script> <script src="/demo/js-header-footer/slow.js"></script> </head> <body> <h1>Javascript in header example</h1> The following is the time difference in milliseconds of these two value: <ul> <li>Time calculated using javascript Date in header immediately after title tag.</li> <li>Time calculated using javascript Date close to the end.</li> </ul> Here javascript is inclued in the header after the first time value is calculated. <br/> <script type="text/javascript"> var currentTime = new Date(); var deltaMillis = currentTime - startTime; document.write("<p>deltaMillis=" + deltaMillis + "<p/>"); </script> </body> </html>You can see the live demo at javascript in header example. Here is the outcome from the above html in Google Chrome
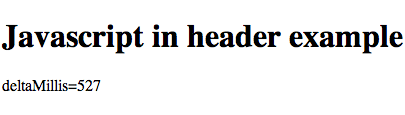
Case 2 – Javascript in footer
Here is the sample code which puts javascript slow.js in html page footer which causes a delay of 500 milliseconds. In this case time difference is calculated and printed before slow.js in html page document.
<!DOCTYPE html> <html> <head> <title>Javascript in footer example</title> <script type="text/javascript"> var startTime = new Date(); </script> </head> <body> <h1>Javascript in footer example</h1> The following is the time difference in milliseconds of these two value: <ul> <li>Time calculated using javascript Date in header immediately after title tag.</li> <li>Time calculated using javascript Date close to the end.</li> </ul> Here javascript is inclued in the footer after the second time value is calculated. <br/> <script type="text/javascript"> var currentTime = new Date(); var deltaMillis = currentTime - startTime; document.write("<p>deltaMillis=" + deltaMillis + "<p/>"); </script> </body> </html> <script src="/demo/js-header-footer/slow.js"></script>You can see the live demo at javascript in footer example. Here is the outcome from the above html in Google Chrome:
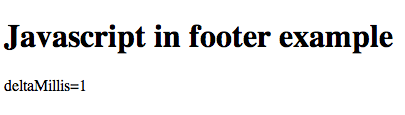
Conclusion
When javascript is placed in the footer, html rendering is faster for the content before the javascript. Also note that the overall execution time of the page should be same in both cases.